UT2004 - Coding Resources - Hit Effects
Unfortunately not implemented by default in UT2004, here is some code for spawning different hit effects and sounds for different surface types intended for use with custom weapons and the like. There are eleven different surface types in UT2004, with room for an extra 32 custom surface types if you're doing a total-conversion.
Here are some screenshots of the effects on a few of the surfaces:
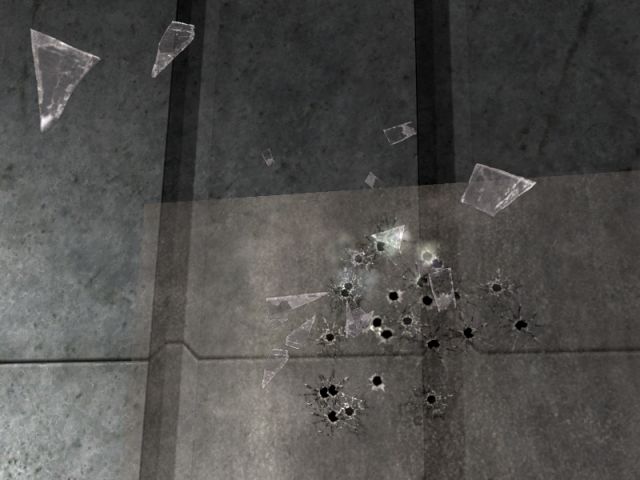
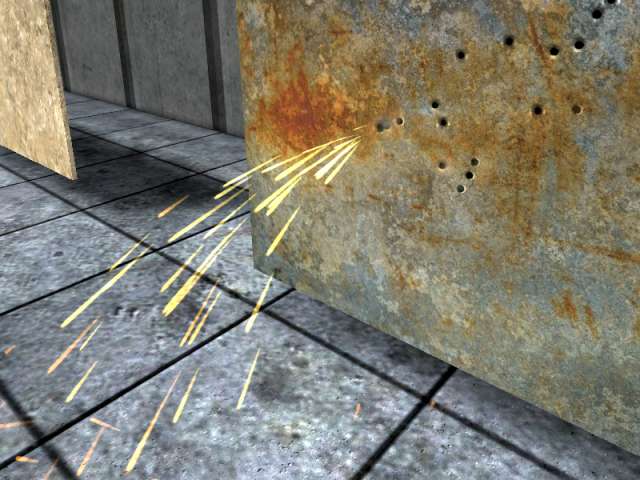
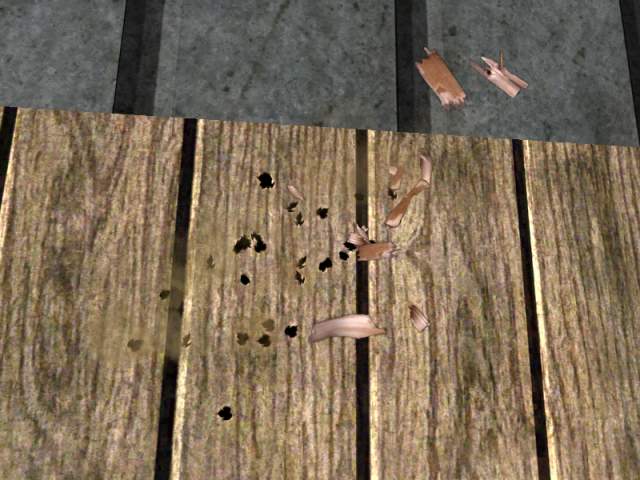
There are three main parts to the code:
- WoEHitEffect: What needs to be spawned to do the effects. It uses a trace to automatically determines what type of surface has been hit, and spawns the appropriate effects. Underwater it spawns just a puff of smoke, although you can of course change that behaviour if you wish. It also has some code for easy precaching of the materials used by the various effects.
- WoEHitEmitter: Spawned by WoEHitEffect. There is one of these for each surface type. Most of them have two emitters in them: one small puff of smoke and one shower of lumps (which is set to high-detail only). This class is also responsible for playing one of a selection of sound effects.
- WoEBulletDecal: A projector to make a hole or dent in the surface. There are several of these for the different surface types, and each one has several different possible textures, chosen at random.
Note that since this code was written with the WoE mod, there are lots of references to WoE packages. Obviously you should name your packages something else to avoid confusion.
In addition to the code there are textures and sounds. You can import these into separate utx and uax packages or compile them into a u file along with the code, whatever your preference is. Note that the decal textures (*Markn.bmp) must be set to TC_Clamp to avoid tiling when projected onto terrain. I'm assuming you know how to do all that, and how to compile the code.
It is very easy to spawn the effects from either an InstantFire trace or from a projectile when it hits something. You need to spawn a WoEHitEffect at the hit location pointing in the direction of the shot, towards the surface. It should be spawned client-side only.
For instance, from a weapon attachment the same as the minigun spawns its boring spark effects:
GetHitInfo();
if(mHitActor != None)
{
Spawn(class'WoEHitEffect',,, mHitLocation, rotator(mHitLocation - (Instigator.Location + Instigator.EyePosition())));
}
or from a projectile:
simulated function HitWall(vector HitNormal, actor Wall)
{
if(Level.NetMode != NM_DedicatedServer)
{
Spawn(class'WoEHitEffect');
}
}
There are other possible uses for this code, such as spawning footprints and dust kicked up from players walking.
Call WoEHitEffect.StaticPrecache if you want to take advantage of the precaching code (otherwise you may get a slight pause when the effects are first displayed as it loads the textures). The most likely place to do this is in a weapon pickup (if it's a weapon that you're using the effects for):
static function StaticPrecache(LevelInfo L)
{
class'WoEHitEffect'.Static.StaticPrecache(L);
}
simulated function UpdatePrecacheMaterials()
{
Super.UpdatePrecacheMaterials();
class'WoEHitEffect'.Static.StaticPrecache(Level);
}
To make it easier to test it all, I've created a small map with a series of panels, one for each material type for shooting at.
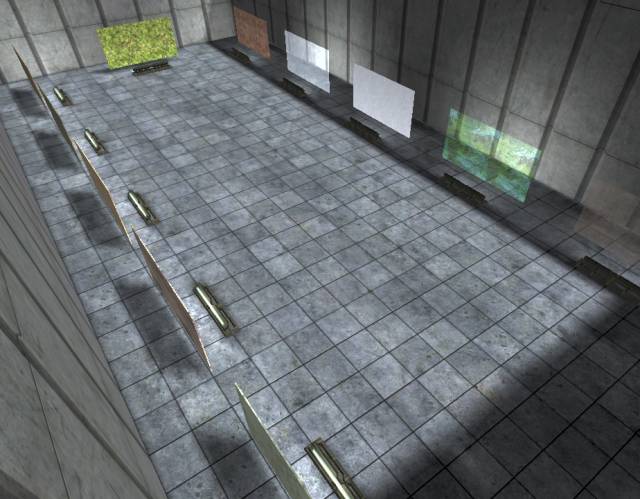
To open it, use the open mapname console command, or double-click on it.
After reading all this way I suppose you want the actual file. Here it is (279KB): HitEffects.zip
That's all there is to it. Some of the sound effects are a bit dodgy, especially the wood hit sounds. If you make use of this code and create better sound effects, please send them my way so I can update this page so everyone can benefit from them. The same applies if you create new and better emitters or decals, or expand or improve the code.